Lab 8 - Images & Tables
Overview
This week's lab will cover the following:
- Creating a new page, called about.html.
- Inserting images into a web page.
- Using tables to format and display content.
- Using CSS to float images.
- Applying CSS to stylize text.
- Positioning HTML elements using CSS.
Lecture Slides
Creating a new page
Create a new page called about.html, that meets the following criteria. Refer to your previous lab instructions if needed.
- Use the HTML5 doctype declaration
- Set the Title to "About Me", without the quotes
- Insert meta tags indicating the encoding (charset) of utf-8, viewport and the author to your name
- Insert a comment indicating your name and student number
- Link your style sheet to the page
- Make use of the main tag, as we did in Lab 6
Modifying your navigation bar
Copy your navigation bar from your index page and paste it at the top of the body, above the <main> section. Use the following steps to modify it to properly indicate your current page.
- Move the class attribute from it's current position (Home) to the link for About
- Modify the hyperlink for Home to direct you to "index.html"
- Modify the hyperlink for About to point to an empty ID ("#")
Inserting a heading
Use the H1 tag to insert a heading with the text "About Me"
Inserting an image
You're going to insert an image of yourself, with a few paragraphs about yourself beside it.
To do:
Create a new folder in your local git folder called images, and copy the picture there. This will be pushed to GitHub when you make a commit through VS Code. Alternatively, you could create the folder and upload your picture directly to GitHub via a web browser.
Insert the image
To insert an image, you are going to use the image tag and provide it with a few parameters. The main ones we'll use are:
- src
- This means source. This can be an absolute or relative path to the image. In this case, it is a relative path.
- alt
- Alternate text that will be displayed in the browser if the image can't be loaded.
Using the following code example, insert the image. Be sure to change file.jpg to match the name and file extension for your image. Ideally your image should not have any spaces in the file name. If it does, they must be represented with %20.
<img src="images/file.jpg">
To do:
Using the paragraph tag, write an introduction paragraph about yourself. Implement an ordered or unordered list (your choice), describing your interests. These can be activities, games, tv shows or movies you watch, etc.
Introducing Tables
Tables consist of several structural tags. They're opened and closed with the <table> and </table> tags respectively. Tables contain 3 main tags for structure.
- <tr> and </tr>
- Specifies a table row.
- <th> and </th>
- Specifies a heading.
- <td> and </td>
- Specifies a cell within the row, or table data.
You are going to insert a table containing your course schedule. This is useful, as you'll be able to access your schedule from your phone as a web page after completion of this lab!
Add an opening table tag, an example follows.
<table>
Start a row, and using the table heading tag specify headings for days of the week. Leave the first cell blank, as that column is going to contain the start time for our classes. Your table should include days for every day Monday-Friday, unlike the sample. If you have Saturday or Sunday classes, include those days too.
<tr> <th></th> <th>Monday</th> <th>Tuesday</th> ... </tr>
Start a new row, and using the table data tag populate your table. The first column is our time, so that should indicate 08:30 - 10:20. You're going to list every class in that time slot, following the sequence outlined in your table header.
<tr> <td>08:30 - 10:20</td> ... </tr>
Times for classes, for reference include:
- 08:30 - 10:20
- 10:30 - 12:20
- 12:30 - 14:20
- 14:30 - 16:20
- 16:30 - 18:20
- 18:30 - 20:20
- 20:30 - 22:20
Once you have your class schedule entered in, close your table.
</table>
If you save and refresh your page, you'll notice the table has inherited the positioning of the list above making it not quite centred. There are a couple different approaches to fix this; the easiest of which is to insert a break tag in between your list and table.
To do:
Insert a break tag between your list and table.
<br>
Resizing and floating the image
Open your stylesheet (style.css). Before you float your image, you should probably resize it. You could open it in Photoshop or Paint (in Windows), but that takes additional time and work. Instead, we can resize the image using CSS. This provides the additional benefit of making the image responsive (or scale with the size of the device it is viewed on). When you're resizing an image you should pick either the width or height to resize, and set the other to auto. You may have to play around with this a bit by making changes and refreshing in your browser. The following code will resize the image. Change the values as needed (since the size of your image might be bigger or smaller than mine).
img { width: 25%; height: auto; }
Next, float the image to the left of the text by adding adding the float parameter to the img selector we just created.
img { float: left; }
Refresh your page. You should notice the text now appears to the right of the image.
Stylizing our table
The first thing you are going to do, is give your table & the cells within a border. You are going to accomplish this using a complex selector.
th, td { }
There are a couple different ways we can add a border. You can use the simpler, longer drawn out method of specifying each of the following:
- border-width: .25em;
- border-style: solid;
- border-color: #000000;
Alternatively, you can use a more complex shorthand to declare the same. The following would apply the exact same specifications as above, note the difference in syntax.
th, td { border: .25em solid #000000; }
Using the above syntax as an example, choose a thickness, style and colour for your border. The styles you have to choose from are:
- none
- hidden
- dotted
- dashed
- solid
- double
- groove
- ridge
- inset
- outset
Feel free to explore the use of the border-radius parameter with any of the table contents (tr, th, td).
Applying some finishing touches
Fixing the paragraph background color
You may notice your background colour from your paragraph appears behind your image. We can remove this by applying a class or id to that particular paragraph, and modify the left margin. You may need to play around with the units to get it to appear in a manor you're satisfied with. First, apply an ID to that paragraph.
<p id="about">
Now using a complex selector, modify the left margin for paragraphs with the id you just created.
p#about { margin-left: 5em; }
Increase or decrease this number as much as needed, until the paragraph background color appears similar to the provided sample (located at the end of the lab instructions).
Centering your table
The easiest way to center a table, is to set the left and right margin to auto. Use the following example to center your table. This same method can be applied to centering many elements.
table { margin-left: auto; margin-right: auto; }
Cleaning up the table formatting
Add line breaks between each course code and room in your table, to make your timetable look nicer.
You may also want to increase the padding on your th & td tags, to make your table look a little nicer. To do that, find the td, th selector we used before and add the padding attribute (sample below). Feel free to play around with the values to get it where you like it.
td, th { padding: .5em; }
Completing the Lab
Using the steps outlined at the end of Lab 7, pull your changes into your AWS instance and take a screenshot. Your page should look similar to the following output. If it does not, go back and complete whatever you've missed.
Your page should validate using the w3schools html validator & css validator. To submit your lab you need to submit the code to your GitHub repo (which you did in already in the lab). Additionally, you need to submit a screenshot of it running your server to the assignment folder in eCentennial.
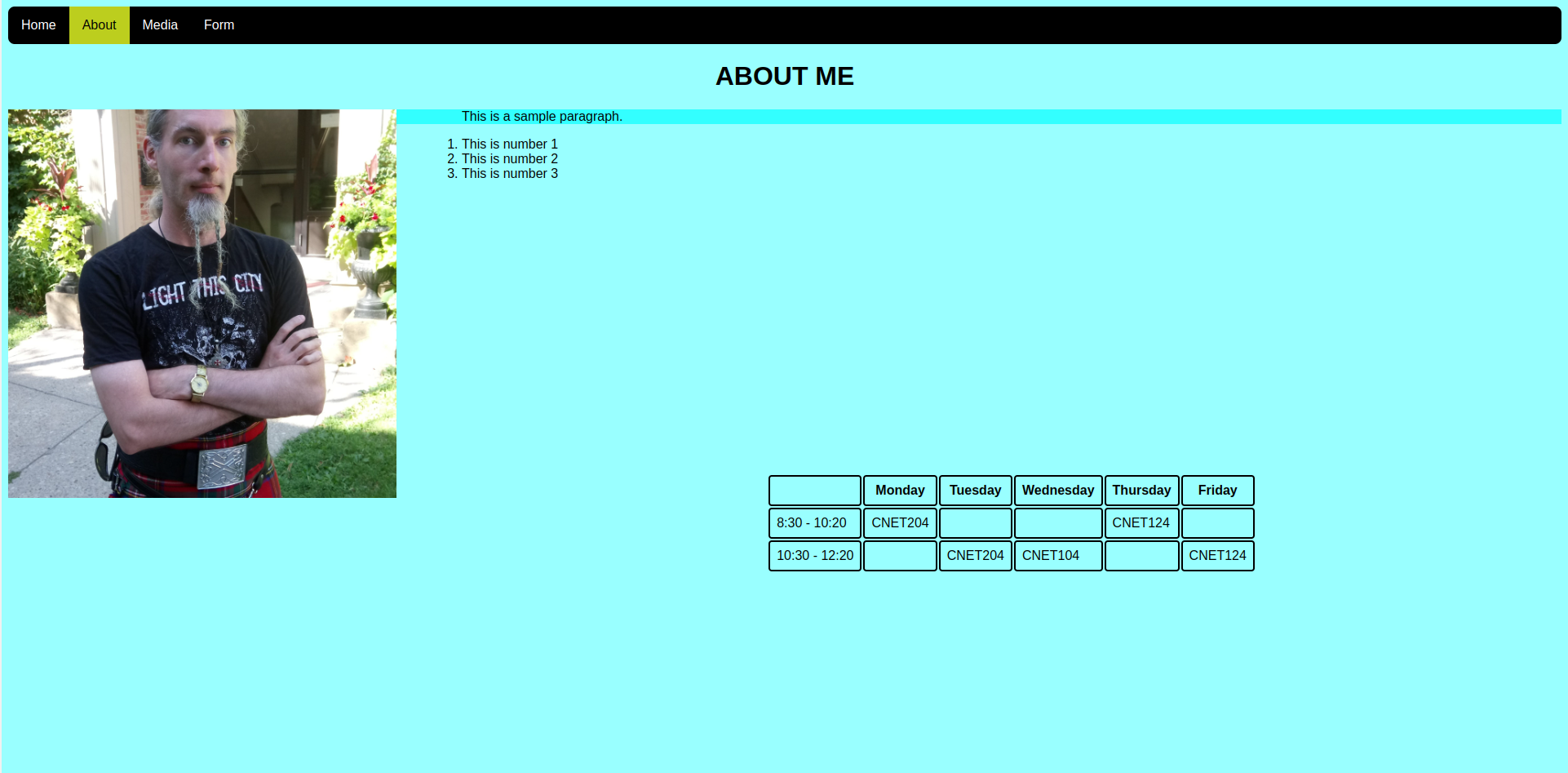
Exploration Questions
- What two tags can table rows contain?
- How do you open and close a table?
- What do the src and alt parameters mean for images?